Project1Fall13
Contents |
Project 1: Applied Linear Algebra
Your project should build on the provided base code. Before you start with this project, you will need to look through the base code and get familiar with it.
This project has three parts, but only the first two are mandatory to get full credit (100 points). The number of points you can get for each part is indicated below. In the first part you will create classes for vectors and matrices. You will use this functionality in the remaining projects throughout the quarter. In the second part of this project you will interactively manipulate the cube from the base code by using keyboard commands.
The third part is optional: you are going to have to tessellate a 3D soccer ball.
This project is due on Friday, October 4th. You will need to present your project in CSE basement labs starting at 1:30pm that day, or during one of the TA/tutors' office hours before the due date. For this project you are allowed to work in teams of two. In this case both team members need to be present during the homework presentation.
TA Matteo Mannino is going to introduce this homework assignment in Center Hall 105 on Monday, September 30th at 3pm.
1. Vectors and Matrices (50 points)
Start with the spinning cube from the base code. Part of the project is a class named Matrix4. Use it as a model and implement matrix and vector classes with frequently used functionality as listed below. Create separate classes for a Vector3 and a Vector4, vectors with three or four components, respectively. The Vector4 type includes the homogeneous coordinate (w component), which helps distinguish points from vectors. There only needs to be a matrix class for 4x4 matrices. As a result of this part of the homework assignment you should have 6 files: Vector3.cpp, Vector3.h, Vector4.cpp, Vector4.h, Matrix4.cpp and Matrix4.h with the following class methods:
1a. Vector3 class (One point for each method for a total of 15 points)
- A constructor with three parameters for the vector coordinates
- Element access 'set': set the vector coordinates
- Element access 'get': return a specific coordinate of the vector
- Overload operator '[]' as alternative to 'get' method
- Vector addition
- Overload operator '+' for addition
- Vector subtraction
- Overload operator '-' for subtraction
- Negation
- Scale (multiplication with scalar value)
- Dot product
- Cross product
- Magnitude (length of vector)
- Normalize
- Print (display the vector's components numerically on the screen)
1b. Vector4 class (One point for each method for a total of 11 points)
- A constructor with three parameters for point coordinates
- A constructor with four parameters
- Element access 'set': set each coordinate separately
- Element access 'get': return one of the four coordinates
- Overload operator '[]' as alternative to 'get' method
- Vector addition
- Overload operator '+' for addition
- Vector subtraction
- Overload operator '-' for subtraction
- Dehomogenize (make fourth component equal to 1)
- Print (display the point's components numerically on the screen)
1c. Matrix4 class (Two points for each method for a total of 24 points)
Implement the following methods in your Matrix4 class:
- Constructor with 16 parameters to set the values of the matrix
- 'get(x,y)' function to read any matrix element
- Multiply (matrix-times-matrix)
- Multiply (matrix-times-vector)
- Make a rotation matrix about the x axis
- Make a rotation matrix about the y axis
- Make a rotation matrix about the z axis
- Make a rotation matrix about an arbitrary (unit) axis
- Make a non-uniform scaling matrix
- Make a translation matrix
- Print the matrix (display all 16 matrix components numerically on the screen in a 4x4 array)
- Transpose the matrix
2. Controlling the Cube (50 points)
Using your vector math classes from part 1, control the cube in various ways with keyboard commands. During this entire time, the cube should keep spinning. The commands should be cumulative, meaning that they build on one another. Never reset the position of the cube or its size, except with the reset command.
Add support for the following keyboard commands to control the cube. You can parse key presses with the glutKeyboardFunc command, for which you can find a tutorial here.
Note that some of the below keyboard commands distinguish between upper and lower case.
- 'c': reverse the direction of the spin. (5 points)
- 'x'/'X': move cube left/right by a small amount. (5 points)
- 'y'/'Y': move cube down/up by a small amount. (5 points)
- 'z'/'Z': move cube into/out of the screen by a small amount. (5 points)
- 'r': reset cube position, orientation, size and color. (5 points)
- With every key press, display the new cube position with your Vector3 print method in the text window. (5 points)
- 'a'/'A': rotate cube about the OpenGL window's z axis by a small number of degrees (e.g., 10) per key press, counterclockwise ('a') or clockwise ('A'). The z axis crosses the screen in the center of the OpenGL window. This rotation should not affect the spin other than that it will rotate the spin axis with the cube. (10 points)
- 's'/'S': scale cube down/up (about its center, not the center of the screen). To scale up means to make it bigger (5 points)
- '1','2','3','4': change the color of the cube's faces to red, green, blue, or yellow, respectively (5 points)
3. Soccer Ball (optional, 10 points)
2014 is the year of the soccer world cup in Brazil. You have been tasked with creating a 3D model of a soccer ball for their national television network.
OpenGL and GLUT offer utility functions to allow you to render simple 3D shapes, such as spheres, cubes and cones. However, a soccer ball shape is not among them, so you will have to generate your own.
To get the optional credit, you will need to implement your own tessellation algorithm for a soccer ball. Tessellation is the representation of a complex shape with many simple shapes, such as triangles. A soccer ball consists of 12 regular pentagons (that are usually painted black) and 20 regular hexagons (painted white). You need to use OpenGL functions to draw a number of triangles that constitute the 3D soccer ball. You need to compute the vertex positions and connect them to triangles. (8 points)
Once you have created the soccer ball shape, you need to color the triangles according to whether they are part of one of the hexagons or pentagons. (1 point)
This web page provides step by step instructions for this process, which can be very helpful.
Once your soccer ball is complete, add it as an alternative shape to your code from part 2. Add a new keyboard command for the 'b' key to toggle between the cube and the soccer ball. Make sure the keyboard commands affect it correctly. You do not need to change the soccer ball's colors. (1 point)
Below is an image of a soccer ball for inspiration:
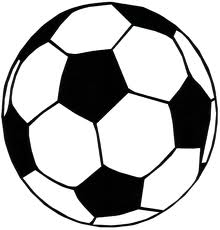
Helpful link: